代码结构优化
一、优化main函数的匿名函数(对函数结构进行优化)
package main
import (
"net/http"
)
func userLogin(writer http.ResponseWriter, request *http.Request) {
//数据库操作
//逻辑处理
//restapi json/xml 返回
//1.获取前端传递的参数
//mobile,passwd
//如何获取参数
//解析参数
request.ParseForm()
mobile := request.PostForm.Get("mobile")
passwd := request.PostForm.Get("passwd")
loginOk := false
if mobile == "15313311315" && passwd == "123456" {
loginOk = true
}
str := `{"code":0,"data":{"id":1,"token":"test"},"msg":"登录成功"}`
if !loginOk {
//返回失败的JSON
str = `{"code":-1,"data":"","msg":"密码不正确"}`
}
//设置header 为JSON 默认的text/html,所以特别指出返回的为application/json
writer.Header().Set("Content-Type", "application/json")
//设置200状态
writer.WriteHeader(http.StatusOK)
//输出
writer.Write([]byte(str))
}
func main() {
//把前端请求的格式和封装处理函数进行绑定的标签
//绑定请求和处理函数
http.HandleFunc("/user/login", userLogin)
//启动web服务器
http.ListenAndServe(":8080", nil)
}
二、优化返回Json代码
package main
import (
"encoding/json"
"log"
"net/http"
)
func userLogin(writer http.ResponseWriter, request *http.Request) {
//数据库操作
//逻辑处理
//restapi json/xml 返回
//1.获取前端传递的参数
//mobile,passwd
//如何获取参数
//解析参数
request.ParseForm()
mobile := request.PostForm.Get("mobile")
passwd := request.PostForm.Get("passwd")
loginOk := false
if mobile == "15313311315" && passwd == "123456" {
loginOk = true
}
if loginOk {
//{"id":1,"token":"xx"}
data := make(map[string]interface{})
data["id"] = 1
data["token"] = "test"
Resp(writer, 0, data, "登录成功")
} else {
Resp(writer, -1, nil, "密码不正确")
}
}
type H struct {
Code int
Msg string
Data interface{}
}
func Resp(w http.ResponseWriter, code int, data interface{}, msg string) {
//设置header 为JSON 默认的text/html,所以特别指出返回的为application/json
w.Header().Set("Content-Type", "application/json")
//设置200状态
w.WriteHeader(http.StatusOK)
//定义一个结构体
h := H{
Code: code,
Msg: msg,
Data: data,
}
//将结构体转化成JSON字符串
ret, err := json.Marshal(h)
if err != nil {
log.Println(err.Error())
}
//输出
w.Write([]byte(ret))
}
func main() {
//把前端请求的格式和封装处理函数进行绑定的标签
//绑定请求和处理函数
http.HandleFunc("/user/login", userLogin)
//启动web服务器
http.ListenAndServe(":8080", nil)
}
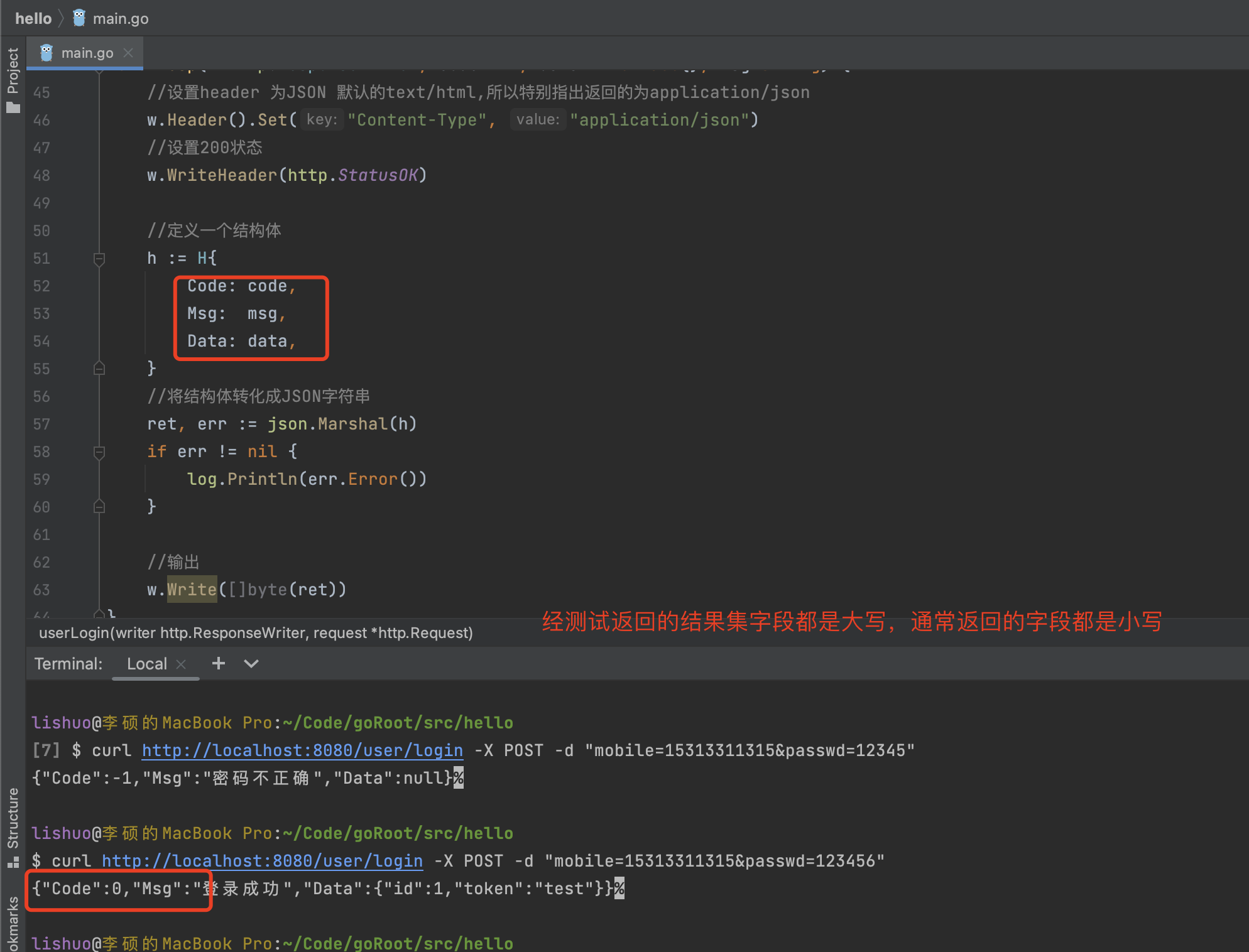
三、优化结构体返回结果集
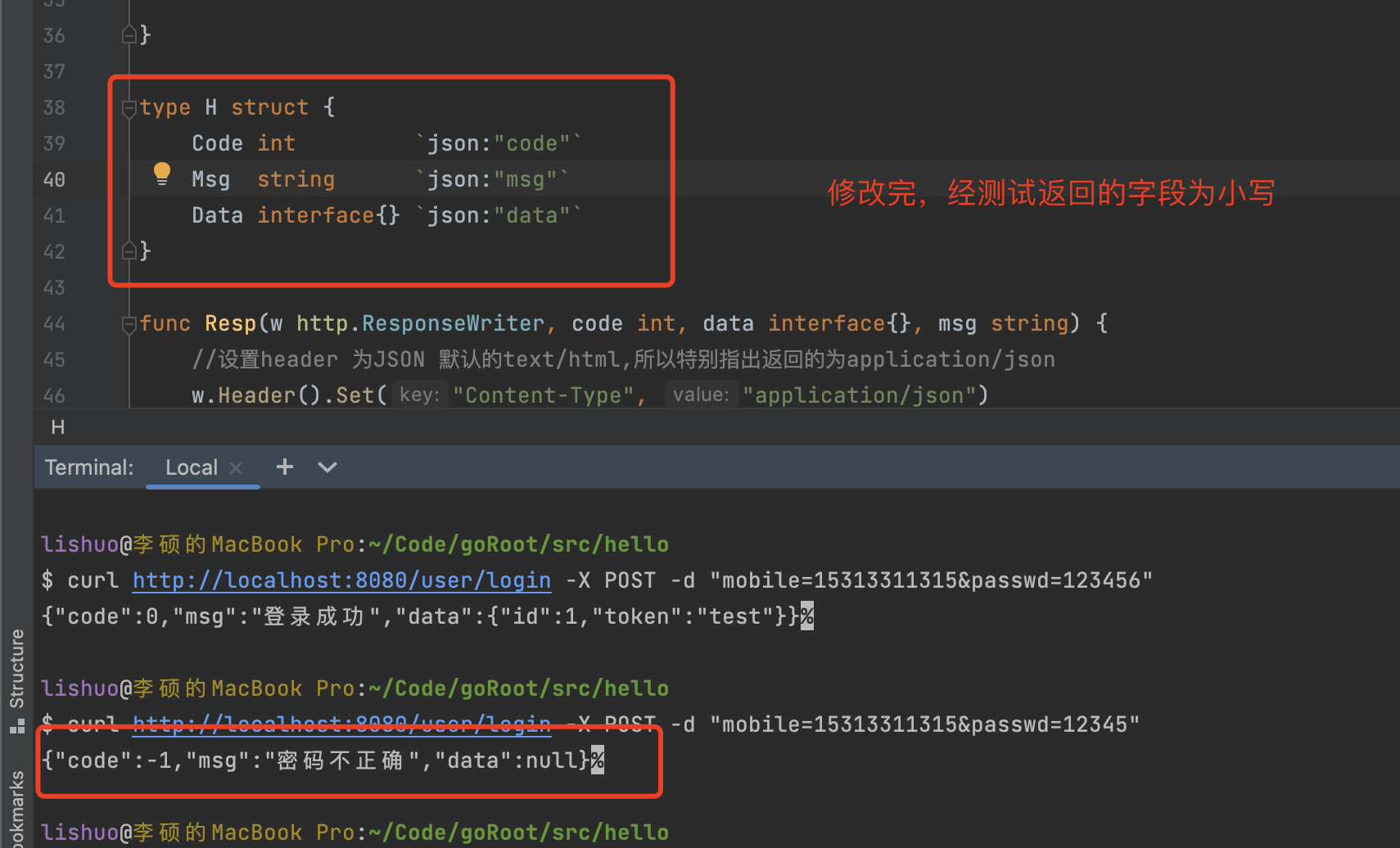
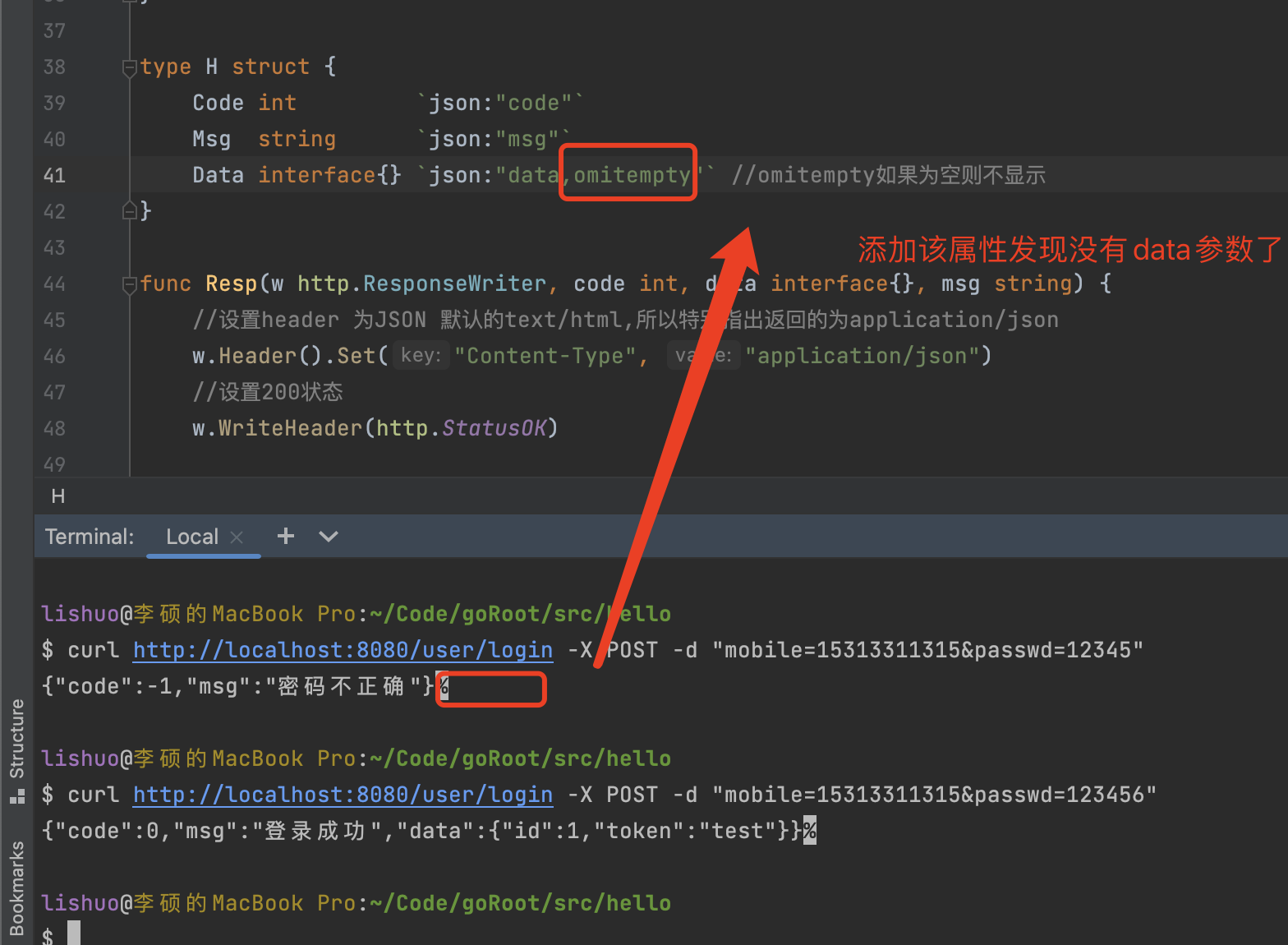