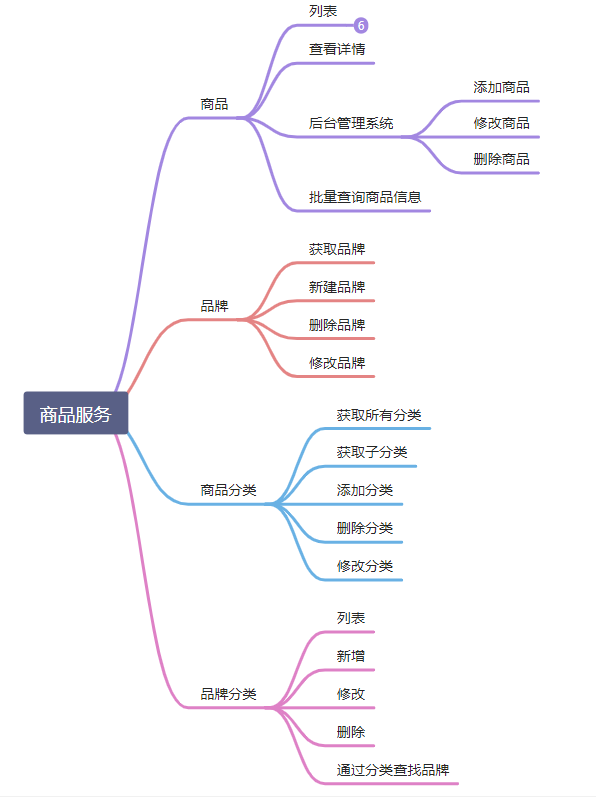
1. 表定义和生成
package model
import (
"database/sql"
"time"
"gorm.io/gorm"
)
type BaseModel struct {
ID int32 `gorm:"primarykey"`
CreateAt time.Time `gorm:"column:add_time"`
UpdateAt sql.NullTime `gorm:"column:update_time"`
DeletedAt gorm.DeletedAt
IsDeleted bool
}
// Category 分类表
type Category struct {
BaseModel
Name string `gorm:"type:varchar(20);not null;comment:分类名称"`
ParentCategoryID int32 `gorm:"type:int(11);default:null;comment:父id 0顶级分类"`
SubCategory []*Category `gorm:"foreignkey:ParentCategoryID;references:ID"`
Level int32 `gorm:"type:int(11);not null;default:1;comment:顶级分类"`
IsTab bool `gorm:"default:false;not null;comment:是否是选项卡"`
}
type Brands struct {
BaseModel
Name string `gorm:"type:varchar(50);not null;comment:品牌名称"`
Logo string `gorm:"type:varchar(255);not null;comment:品牌logo"`
}
type GoodsCategoryBrand struct {
BaseModel
CategoryID int32 `gorm:"type:int(11);not null;comment:商品分类id"`
Category Category `gorm:"foreignKey:CategoryID;references:ID"`
BrandsID int32 `gorm:"type:int(11);not null;comment:品牌id"`
Brand Brands `gorm:"foreignKey:BrandsID;references:ID"`
}
func (GoodsCategoryBrand) TableName() string {
return "goodscategorybrand"
}
// Banner 轮播图表
type Banner struct {
BaseModel
Image string `gorm:"type:varchar(255);not null;comment:图片地址"`
Url string `gorm:"type:varchar(255);not null;comment:跳转地址"`
Index int32 `gorm:"type:int(11);not null;comment:轮播顺序"`
}
type Goods struct {
BaseModel
CategoryID int32 `gorm:"type:int;not null;comment:商品分类id"`
Category Category `gorm:"foreignKey:CategoryID;references:ID"`
BrandsID int32 `gorm:"type:int;not null;comment:品牌id"`
Brands Brands `gorm:"foreignKey:BrandsID;references:ID"`
OnSale bool `gorm:"default:false;not null;comment:是否上架"`
ShipFree bool `gorm:"default:false;not null;comment:是否免运费"`
IsNew bool `gorm:"default:false;not null;comment:是否是新品"`
IsHot bool `gorm:"default:false;not null;comment:是否是热卖"`
Name string `gorm:"type:varchar(100);not null;comment:商品名称"`
GoodsSn string `gorm:"type:varchar(50);not null;comment:商品货号"`
ClickNum int32 `gorm:"type:int;default:0;not null;comment:点击数"`
SoldNum int32 `gorm:"type:int;default:0;not null;comment:销售数"`
FavNum int32 `gorm:"type:int;default:0;not null;comment:收藏数"`
MarketPrice float32 `gorm:"not null;comment:市场价"`
ShopPrice float32 `gorm:"not null;comment:本店价"`
GoodsBrief string `gorm:"type:varchar(100);not null;comment:商品简介"`
Images GormList `gorm:"type:varchar(1000);not null;comment:商品图片"`
DescImages GormList `gorm:"type:varchar(4000);not null;comment:商品详情图片"` // 修改长度
GoodsFrontImage string `gorm:"type:varchar(200);not null;comment:商品封面图"`
}
2. 导入数据
mxshop_goods_srv.sql